Why use the useRef hook instead of useState?
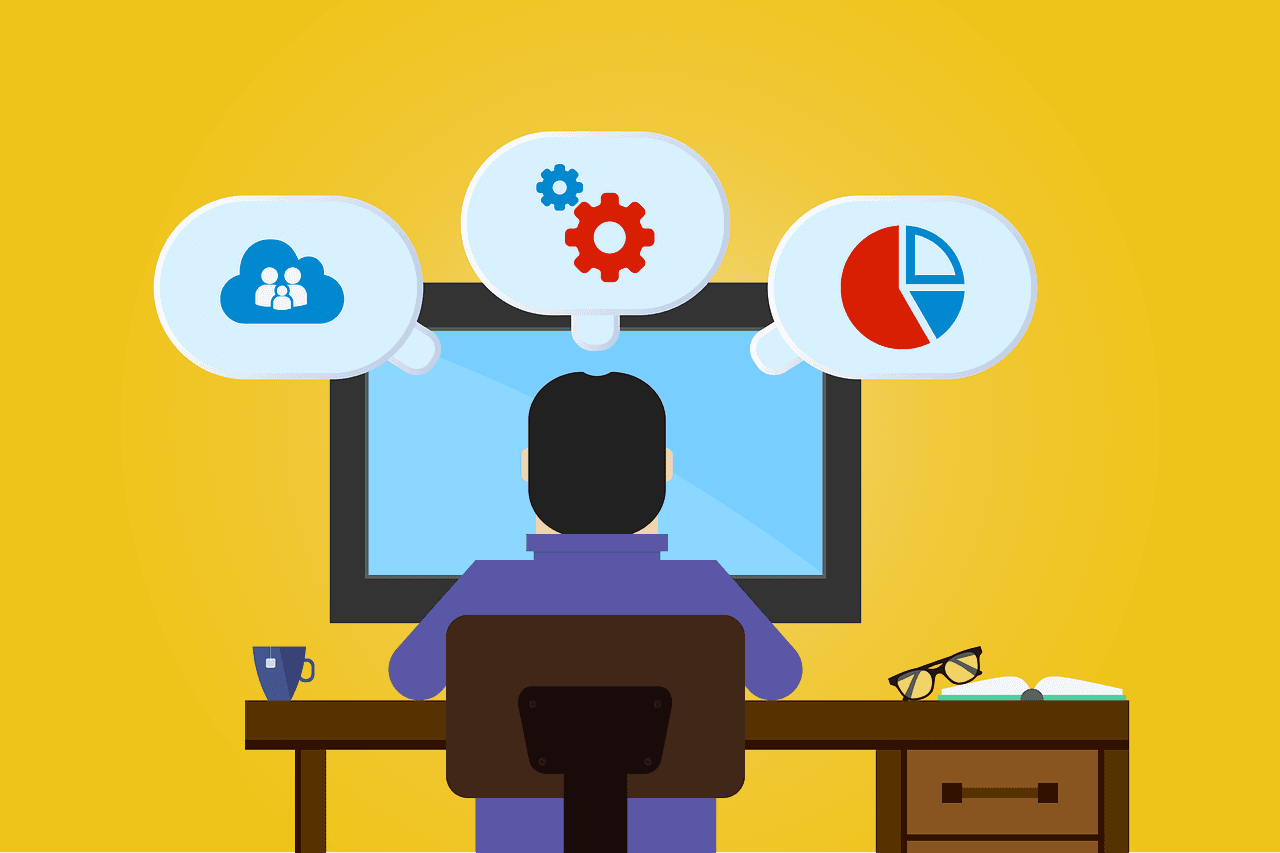
Source: www.pixabay.com
Introduction
In this tutorial we'll be explaining the React useRef
with examples.
The useRef
Hook is a built-in React Hooks that accepts one argument as the initial value and returns a reference (aka ref). It creates a reference to an element or value in a component and returns a ref
object with a .current
property. The main use case of a useRef
hook is for accessing and manipulating a child component or DOM element directly, or creating and storing mutable values across component renders without triggering a re-render.
Let’s get started!
Pre-requisite:
Basic knowledge of Javascript and React is required
The useRef
hook
Example #1: This example shows how to use useRef
without triggering a component re-rendering
import React, { useRef, useEffect } from 'react';
const App = () => {
const inputValueRef = useRef(null);
const handleInputValueRef = () => {
inputValueRef.current.focus();
};
return (
<div>
<input ref={inputValueRef} type="text" />
<button onClick={handleInputValueRef}>Focus Input Field</button>
</div>
);
}
In this example, we created a ref using useRef
and assign it to the inputValueRef
variable. We then attach the ref to the input element using the ref attribute. Also, we added a button and attached a click event with handleInputValueRef
method to it. When the button is clicked, the handleInputValueRef
function is invoked, which uses the inputValueRef.current
to access the DOM node and call the focus()
method on it.
Inside the handleInputValueRef
function, we access the DOM node of the input element by using inputValueRef.current
. The current
property of the ref
object holds the reference to the DOM node. In this case, we use it to invoke the focus()
method on the input element, which sets the focus on the input field.
So, when the button is clicked, the handleInputValueRef
function is called, and it uses the inputValueRef.current
to access the DOM node of the input element and invoke the focus()
method on it, which sets the focus on the input field.
Example #2: Here's another example of how use the useRef
to set the focus on the input field when the component mounts
import React, { useRef, useEffect } from 'react';
const App = () => {
const searchQueryRef = useRef(null);
useEffect(() => {
searchQueryRef.current.focus();
},[]);
return (
<div>
<span>Enter search query:</span>
<input ref={searchQueryRef} type="text" />
</div>
);
}
In this example, we refactored the previous example and placed the
searchQueryRef.current.focus()
within the useEffect
hook which dependencies array is an empty array [], meaning the effect will only run once, when the component mounts. The DOM node of the input element is accessed using searchQueryRef.current
. Then we invoke the .focus()
method on the DOM node which means the input field will receive focus when the component mounts
and ensures that the input field is ready for user input as soon as the component is rendered which could improve the user experience of the functionality.
Further Explanation
As shown in the examples above, the useRef
hook offers advantages over the useState
hook like persisting values across renders and modifying the value of a useRef
hook variable does not trigger a component re-render. This makes it particularly useful in some specific scenarios, such as automatically focusing on an input field to enhance the user experience, and storing the previous state variable, and enabling comparisons between the current and previous values without triggering re-renders. It's important to note that while a "normal" variable wouldn’t cause a re-render, but it wouldn't persist across renders as the component function is executed again, resulting in variable re-initialization.
Summary
In summary, the primary use case of useRef
hook is to persist values across renders without causing unnecessary updates, or manipulating DOM element directly which would improve the user experience of the functionality. Best practice is to use useRef
hook for scenarios when there is no need to re-render the component when the state changes, and useState
for when re-render the component is needed when the state changes.
I hope this helps! Go to the contact page and let me know if you have any further questions.
Happy coding!