How to Build a Simple Toggle values application in React
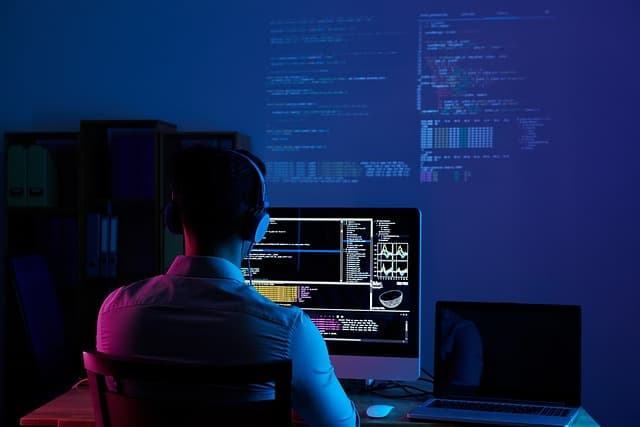
Source: www.pixabay.com
Introduction
This tutorial demonstrates how to build a simple toggle values application in React using HTML Radio buttons control. Let's imagine we want to display two categories of items to users but we would like to show a category at a time. We can use radio buttons to implement a toggle to switch between the values. Let’s get started.
Pre-requisite:
- Basic knowledge of Javascript and React is required
- Proficient in the use of any modern code editors i.e. VS Code
Step #1
-
Scaffold a React application. One quick and easy way to do this is to use the Create React App tool. See Create React App site for details
-
Create a React app project and give it the name - toggle-values
npx create-react-app toggle-values
Step #2
- Open the toggle-values project in any code editor of your choice
- Open the App.js file and delete the content in the return statement.
Step #3
- import
useState
in the first line
import React, { useState } from "react";
- Declare a state property , a setter using the
useState
hook and give it a default value oftrue
const [isToggle, setIsToggle] = useState(true);`
Step #4
- Create a change method and use the setter method -
setIsToggle
to set theisToggle
value
const toggleChange = ()=>{
setIsToggle(!isToggle)
}
FYI: The exclamation mark !
is a logical “not” operator. It inverts a boolean expression in JavaScript. For example, if a value is true
it will invert to a false
vice visa.
Step #5
- Add two Radio buttons, and set the attribute as shown below.
<div>
<input
type="radio"
value={true}
name="toggle"
checked={isToggle}
onChange={toggleChange}
/>
<label>Yes </label>
<input
type="radio"
value={false}
name="toggle"
onChange={toggleChange}
/>
<label>No </label>
</div>
Step #6
- Create the two values and use
isToggle
as the ternary operator condition to determine what would be displayed. See below:
{
isToggle ? (<h1> Yes Items</h1>):
(<h1> No Items</h1>)`
}
Complete code for the toggle values implementation
import React, { useState } from "react";
export default function App() {
const [isToggle, setIsToggle] = useState(true);
const toggleChange = ()=>{
setIsToggle(!isToggle)
}
return (
<>
<h1>Simple Toggle Values </h1>
<div>
<input
type="radio"
value={true}
name="toggle"
checked={isToggle}
onChange={toggleChange}
/>
<label>Yes </label>
<input
type="radio"
value={false}
name="toggle"
onChange={toggleChange}
/>
<label>No </label>
</div>
{
isToggle ? (<h1> Yes values</h1>):
(<h1> No values</h1>)
}
</>
);
}
Live demo: How to build a simple toggle values in React!:-
That's it. Happy coding!